資料介紹
描述
此 Arduino 線性致動器教程展示了如何使用 Arduino 兼容板和各種輸入傳感器控制Firgelli 小型線性致動器,包括用于直接控制的滑塊和旋轉旋鈕、用于增量移動的操縱桿以及具有預設位置的三個按鈕(在代碼中預設)每個位置都分配給一個按鈕,因此當用戶按下按鈕時,小型線性執行器會移動到該位置)。
對于 Arduino 線性執行器項目,Firgelli 的小型線性執行器非常出色。這些線性執行器有一個內部控制器,允許它們像伺服一樣操作。通過使用 Arduino 伺服庫,我們可以簡單地向執行器發送所需的位置,然后它移動到該位置。
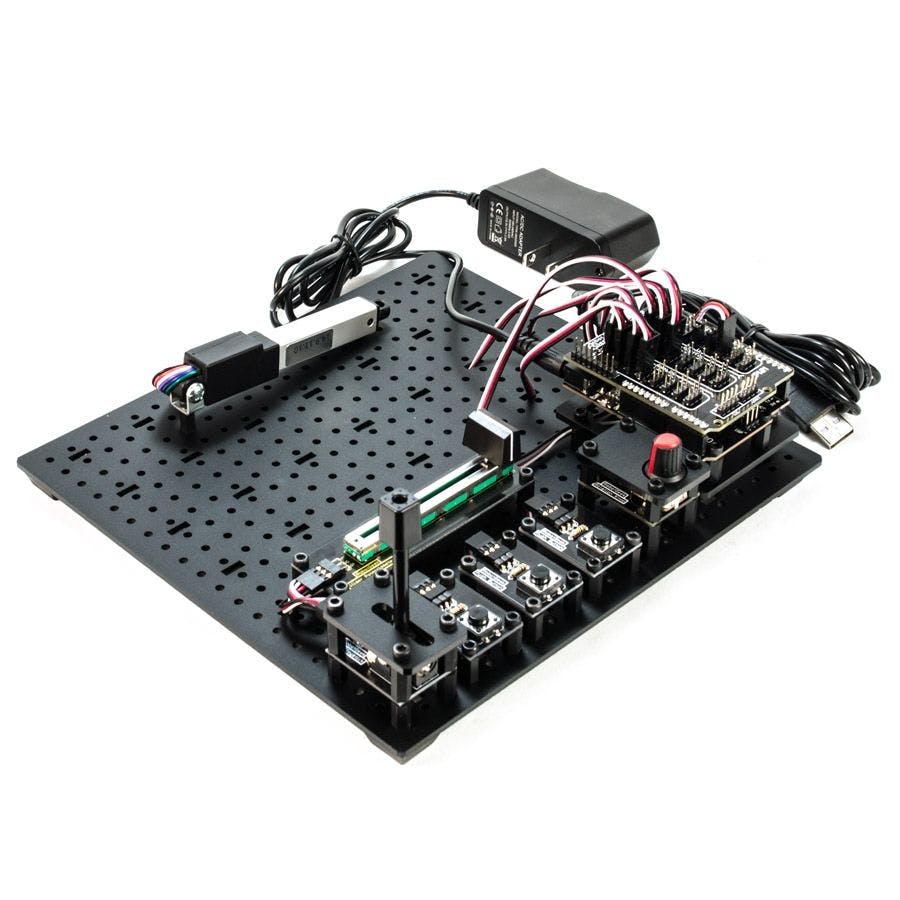
所有部件都可以在RobotGeek Arduino 線性執行器實驗者套件中一起找到,或者單獨獲得。
第 1 步:接線
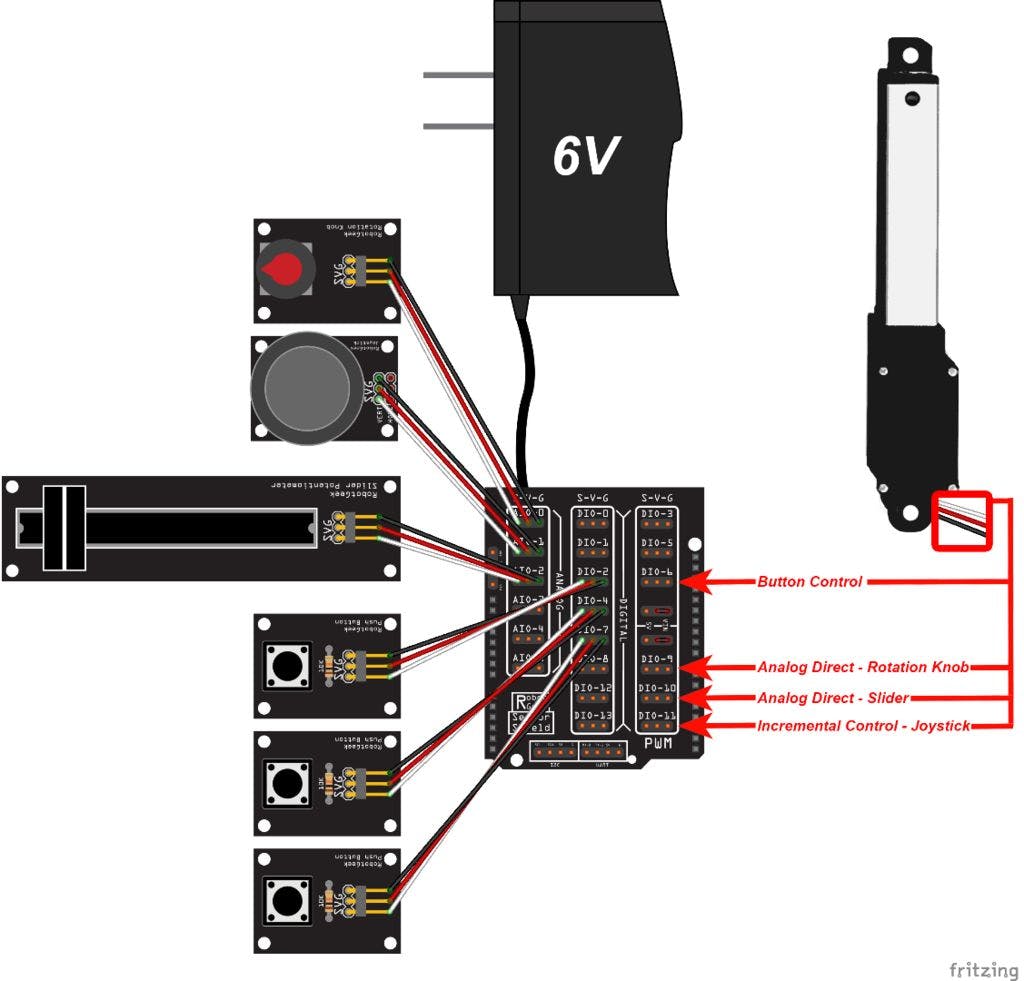
?
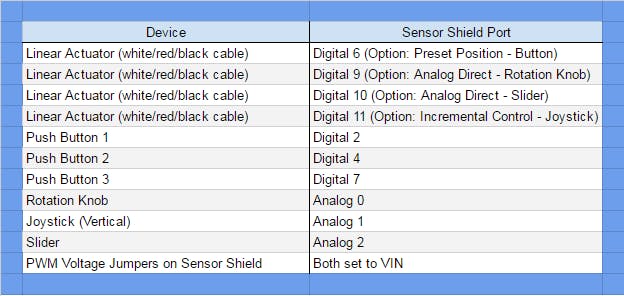
請注意,您必須將線性致動器物理插入不同的端口才能使用不同的控件移動它。如果您有多個線性執行器,您可以使用此代碼同時控制多達 4 個。
第 2 步:將代碼上傳到您的 Arduino
您可以在RobotGeek 庫和工具中的以下位置找到此演示代碼:
RobotGeekSketches -> Demos -> LinearActuator -> linearActuatorExpDemo -> linearActuatorExpDemo.ino
這段代碼將幫助我們完成接下來的三個部分。根據您正在學習的教程部分,您將被要求將線性致動器插入不同的引腳。
/***********************************************************************************
* RobotGeek Linear Actuator Experimenter's Kit Demo
* ________
* | \---------\___________________________
* __| | ||--------------------|__
* (o | FIRGELLI | o ||____________________| o)
* |__________________/--------------------------||
*
*
* The following sketch will allow you to control a Firgelli Linear actuator using
* the RobotGeek Slider, RobotGeek Knob, RobotGeek Joystick, and RobotGeek Pushbuttons
*
* http://www.robotgeek.com/robotgeek-experimenters-kit-linear-actuator
*
*
* Wiring
* Linear Actuator - Digital Pin 6, 9, 10, and/or 11
*
* Knob - Analog Pin 0
* Joystick - Analog Pin 1
* Slider - Analog Pin 2
*
* Pushbutton - Digital Pin 2
* Pushbutton - Digital Pin 4
* Pushbutton - Digital Pin 7
*
* Jumpers for pins 3/5/6 and 9/10/11 should be set to 'VIN'
*
*
* Control Behavior:
* Moving the slider or knob will move the linear actuator keeping absolute position.
* Moving the joystick will move the linear actuator incrementally.
* Pressing one of the buttons will move the linear actuator to a predetermined position.
*
***********************************************************************************/
//Includes
#include //Servo Library can be used for Firgelli Mini Linear Actuators
//Linear Actuator Pins
const int LINEARPIN_BUTTON = 6; //Linear Actuator on Digital Pin 6
const int LINEARPIN_KNOB = 9; //Linear Actuator on Digital Pin 9
const int LINEARPIN_SLIDER = 10; //Linear Actuator on Digital Pin 10
const int LINEARPIN_JOYSTICK = 11; //Linear Actuator on Digital Pin 11
//Analog Input Pins
const int KNOB_PIN = 0; //Knob on Analog Pin 0
const int JOYSTICK_PIN = 1; //Joystick (vertical) on Analog Pin 1
const int SLIDER_PIN = 2; //Slider on Analog Pin 2
//Digital Input Pins
const int BUTTON1_PIN = 2; //Button 1 on Digital Pin 2
const int BUTTON2_PIN = 4; //Button 2 on Digital Pin 4
const int BUTTON3_PIN = 7; //Button 3 on Digital Pin 7
//Generic deadband limits - not all joystics will center at 512, so these limits remove 'drift' from joysticks that are off-center.
const int DEADBAND_LOW = 482; //decrease this value if drift occurs, increase it to increase sensitivity around the center position
const int DEADBAND_HIGH = 542; //increase this value if drift occurs, decrease it to increase sensitivity around the center position
//Max/min pulse values in microseconds for the linear actuators
const int LINEAR_MIN = 1050;
const int LINEAR_MAX = 2000;
// variables will change:
int button1State = 0; // variable for reading the pushbutton status
int button2State = 0; // variable for reading the pushbutton status
int button3State = 0; // variable for reading the pushbutton status
Servo linearKnob, linearSlider, linearButton, linearJoystick; // create servo objects to control the linear actuators
int knobValue, sliderValue, joystickValue; //variables to hold the last reading from the analog pins. The value will be between 0 and 1023
int valueMapped; // the joystick values will be changed (or 'mapped') to new values to be sent to the linear actuator.
//variables for current positional value being sent to the linear actuator.
int linearValue_Knob = 1500;
int linearValue_Slider = 1500;
int linearValue_Button = 1500;
int linearValue_Joystick = 1500;
int speed = 2;
void setup()
{
//initialize linear actuators as servo objects
linearKnob.attach(LINEARPIN_KNOB); // attaches/activates the linear actuator as a servo object
linearSlider.attach(LINEARPIN_SLIDER); // attaches/activates the linear actuator as a servo object
linearButton.attach(LINEARPIN_BUTTON); // attaches/activates the linear actuator as a servo object
linearJoystick.attach(LINEARPIN_JOYSTICK); // attaches/activates the linear actuator as a servo object
//Analog pins do not need to be initialized
//use the writeMicroseconds to set the linear actuators to their default positions
linearKnob.writeMicroseconds(linearValue_Knob);
linearSlider.writeMicroseconds(linearValue_Slider);
linearButton.writeMicroseconds(linearValue_Button);
linearJoystick.writeMicroseconds(linearValue_Joystick);
}
void loop()
{
//Preset Positions for Button Control
// if the pushbutton is pressed set the linear value
button1State = digitalRead(BUTTON1_PIN);
if (button1State == HIGH) {
// set the position value
linearValue_Button = 1300;
}
button2State = digitalRead(BUTTON2_PIN);
if (button2State == HIGH) {
// set the position value
linearValue_Button = 1500;
}
button3State = digitalRead(BUTTON3_PIN);
if (button3State == HIGH) {
// set the position value
linearValue_Button = 1700;
}
//Analog Direct Control
//read the values from the analog sensors
knobValue = analogRead(KNOB_PIN);
sliderValue = analogRead(SLIDER_PIN);
linearValue_Knob = map(knobValue, 0, 1023, LINEAR_MAX, LINEAR_MIN); //Map analog value from the sensor to the linear actuator
linearValue_Slider = map(sliderValue, 0, 1023, LINEAR_MAX, LINEAR_MIN); //Map analog value from the sensor to the linear actuator
//Incremental Joystick Control
joystickValue = analogRead(JOYSTICK_PIN); //read the values from the joystick
//only update if the joystick is outside the deadzone (i.e. moved oustide the center position)
if(joystickValue > DEADBAND_HIGH || joystickValue < DEADBAND_LOW)
{
valueMapped = map(joystickValue, 0, 1023, speed, -speed); //Map analog value from native joystick value (0 to 1023) to incremental change (speed to -speed).
linearValue_Joystick = linearValue_Joystick + valueMapped; //add mapped joystick value to present Value
linearValue_Joystick = constrain(linearValue_Joystick, LINEAR_MIN, LINEAR_MAX); //
}
//Use the writeMicroseconds to set the linear actuator to its new position
linearKnob.writeMicroseconds(linearValue_Knob);
linearSlider.writeMicroseconds(linearValue_Slider);
linearButton.writeMicroseconds(linearValue_Button);
linearJoystick.writeMicroseconds(linearValue_Joystick);
delay(10);
}
第 3 步:模擬直接控制
對于本節:
要使用旋轉旋鈕,請將線性執行器插入Digital Pin 9
要使用滑塊,請將線性執行器插入Digital Pin 10
那么這是怎么回事?我們正在將模擬傳感器的絕對位置映射到執行器位置。這是有道理的,您將旋轉旋鈕或滑塊移動到一個位置,并且線性執行器與該位置匹配。
想看廢話嗎?將操縱桿插入Analog Pin 0
,將線性執行器插入Digital Pin 9
。操縱桿始終返回到中心位置,導致線性執行器匹配并返回到其中心值。如果您想使用操縱桿將線性致動器移動到中心以外的位置,那么這并不是那么有用,這將我們帶到下一節。在繼續之前,請確保將傳感器恢復到原來的引腳。
如果您想要僅涵蓋本教程這一部分的草圖,可以在此處找到它。
第 4 步:增量控制
對于本節:
要使用操縱桿,請將線性執行器插入Digital Pin 11
那么這是怎么回事?這一次,我們不是直接映射到操縱桿的值,而是使用操縱桿來增加線性致動器的位置,這樣我們就可以放開操縱桿,讓線性致動器保持在上次放置的位置。
如果您想要僅涵蓋本教程這一部分的草圖,可以在此處找到它。
第 5 步:預設控件
對于本節:
要使用按鈕,請將線性執行器插入Digital Pin 6
那么這是怎么回事?在這一部分中,我們使用按鈕按下將線性執行器發送到預定義的位置。當您知道在輸入定義的情況下您希望線性致動器處于什么位置時,這非常簡單且非常有用。
如果您想要僅涵蓋本教程這一部分的草圖,可以在此處找到它。
第 6 步:下一步是什么?
現在您知道了三種控制直線推桿的方法。你能想到可以從中受益的項目嗎?您有想要自動打開的盒子嗎?您將如何使用線性致動器來做到這一點?人體的所有肌肉都是生物線性致動器。你能做一個模仿這個的機械臂嗎?做一張可以傾斜的桌子怎么樣?我們很想聽聽您的項目!去創造吧!
- BeagleBone Black Wireless、MotorCape和線性執行器
- 從單個Arduino輸出引腳控制多個繼電器或其他執行器
- 怎么樣設計機器人的末端執行器 6次下載
- 汽車控制系統的基本介紹,包括傳感器輸入,控制器及執行器詳細概述 32次下載
- 歐瑪部分回轉電動執行器SG05.1–SG12.1使用說明 22次下載
- 電動執行器的功能及應用 19次下載
- 具有執行器飽和與隨機非線性擾動的離散系統模型預測控制_石宇靜 0次下載
- 控制儀表及計算機控制裝置--執行器理論知識 0次下載
- 基于LPC2132的電動執行器雙核控制系統的設計 49次下載
- 執行器的選擇
- 基于HART協議的智能執行器接口卡的開發
- 基于神經網絡的電動執行器狀態診斷
- 基于現場總線的智能執行器控制網絡
- 基于單片機的電動執行器控制系統的開發
- 全數字電動執行器的開發與應用
- 機電執行器概述和演變 948次閱讀
- 智能變頻電動執行器的電流檢測電路介紹 3970次閱讀
- 多層壓電執行器的應用 1499次閱讀
- 使用DAC精確控制線性執行器 2017次閱讀
- 電動執行器的結構原理和常見故障分析 1.6w次閱讀
- 觸覺反饋設計中常用的執行器 2333次閱讀
- 如何使用Arduino控制大型線性執行器 2166次閱讀
- 氣動執行器的組成_氣動執行器選型 4072次閱讀
- 氣動執行器的常見故障和解決方法 7540次閱讀
- 執行器故障原因及檢修 1w次閱讀
- 執行器由什么組成_執行器的工作原理 1.1w次閱讀
- 執行器是什么_執行器的主要作用 1.7w次閱讀
- 電熱執行器的作用 1.3w次閱讀
- 電熱執行器是什么_電熱執行器工作原理 1.9w次閱讀
- 汽車控制系統中的電子控制單元和傳感器以及執行器 9069次閱讀
下載排行
本周
- 1A7159和A7139射頻芯片的資料免費下載
- 0.20 MB | 55次下載 | 5 積分
- 2PIC12F629/675 數據手冊免費下載
- 2.38 MB | 36次下載 | 5 積分
- 3PIC16F716 數據手冊免費下載
- 2.35 MB | 18次下載 | 5 積分
- 4dsPIC33EDV64MC205電機控制開發板用戶指南
- 5.78MB | 8次下載 | 免費
- 5STC15系列常用寄存器匯總免費下載
- 1.60 MB | 7次下載 | 5 積分
- 6模擬電路仿真實現
- 2.94MB | 4次下載 | 免費
- 7PCB圖繪制實例操作
- 2.92MB | 2次下載 | 免費
- 8零死角玩轉STM32F103—指南者
- 26.78 MB | 1次下載 | 1 積分
本月
- 1ADI高性能電源管理解決方案
- 2.43 MB | 452次下載 | 免費
- 2免費開源CC3D飛控資料(電路圖&PCB源文件、BOM、
- 5.67 MB | 141次下載 | 1 積分
- 3基于STM32單片機智能手環心率計步器體溫顯示設計
- 0.10 MB | 137次下載 | 免費
- 4A7159和A7139射頻芯片的資料免費下載
- 0.20 MB | 55次下載 | 5 積分
- 5PIC12F629/675 數據手冊免費下載
- 2.38 MB | 36次下載 | 5 積分
- 6如何正確測試電源的紋波
- 0.36 MB | 19次下載 | 免費
- 7PIC16F716 數據手冊免費下載
- 2.35 MB | 18次下載 | 5 積分
- 8Q/SQR E8-4-2024乘用車電子電器零部件及子系統EMC試驗方法及要求
- 1.97 MB | 8次下載 | 10 積分
總榜
- 1matlab軟件下載入口
- 未知 | 935121次下載 | 10 積分
- 2開源硬件-PMP21529.1-4 開關降壓/升壓雙向直流/直流轉換器 PCB layout 設計
- 1.48MB | 420062次下載 | 10 積分
- 3Altium DXP2002下載入口
- 未知 | 233088次下載 | 10 積分
- 4電路仿真軟件multisim 10.0免費下載
- 340992 | 191367次下載 | 10 積分
- 5十天學會AVR單片機與C語言視頻教程 下載
- 158M | 183335次下載 | 10 積分
- 6labview8.5下載
- 未知 | 81581次下載 | 10 積分
- 7Keil工具MDK-Arm免費下載
- 0.02 MB | 73810次下載 | 10 積分
- 8LabVIEW 8.6下載
- 未知 | 65988次下載 | 10 積分
評論